These days I recommend using the Genesis Framework for WordPress and in the sites I build I incorporate the new Bootstrap v4 grid system into that.
When building yourself a shiny new website it’s often quicker and easier to start off with a template of one that looks similar to what you want – and then modify that. There’s lots of free ones available, and you can get premium ones at places like Themeforest for about £15 that are well coded and commented. There’s often a standard html template version and a WordPress version of the same theme, but the WordPress version is usually twice the price.
Whilst purchasing a ready-made WordPress version has a lot of convenience built in, there’s a lot to be said for rolling your own WordPress theme so you know exactly how it works. That way it’s much easier for you to customise it just how you want it.
Level of knowledge assumed: This tutorial is aimed at the eager amateur. You don’t need to know a lot about how WordPress works but it’s good if you know some basic HTML and CSS and are familiar with editing files and saving them onto your web server using a FTP program like Filezilla.
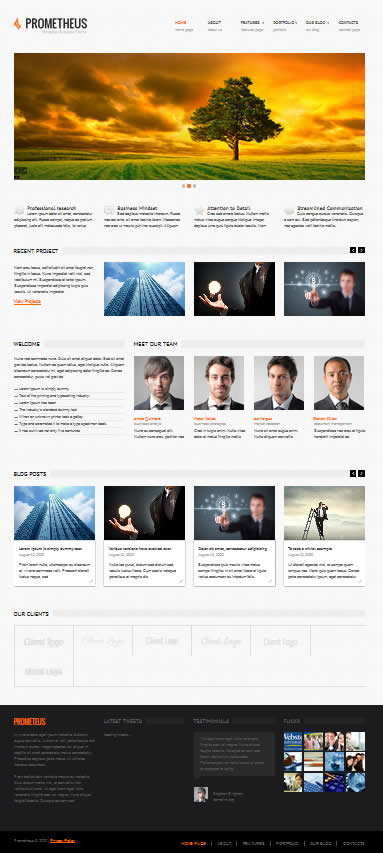
In this tutorial we’ll start with this Bootstrap based theme that I picked up cheap in a Mighty Deals sale. It contains the html, css and js files but not any WordPress code. By the end of the article we’ll have customised it for a small charity, converted it into a basic WordPress theme and plugged it into a new installation of WordPress. You can see the end result here. This process works just the same with a non-Bootstrap template too.
Included in the template from Flashmint (and similar with most others) I’ve got a Photoshop file (that I won’t be needing) and a HTML, a JS and a CSS folder. The HTML folder has templates for all the different pages shown in its demo site. The JS folder has the Bootstrap javascript files plus 10 or so other jquery ones that control the carousel, the Twitter integration, the scroll-to-top button etc. Finally the CSS folder has the basic Bootstrap style sheets plus a couple of custom ones for the styling of this template.
Here’s how we’ll do it:
- Set up a fresh WordPress installation and some dummy content
- Modify the style sheet to register your new WordPress theme
- We’ll take the index.html version (the Home page of the html theme) and turn it into our first WordPress theme template – home.php
- Start with the header
- Convert the footer into WordPress widgets
- Load (enqueue) the javascript and jquery files the proper WordPress way
- Understanding the WordPress Loop
- Using the Loop to display latest/featured posts
- The content sliders and carousels
- The complicated bit – the menu and Bootstrap walker
- The single post template
- The sidebar
- Archive/category pages
- Other WordPress templates (404, search etc).
- Bootstrap shortcodes
- Next steps/troubleshooting
Ready to get stuck in? Let’s go…
1. Set up a fresh WordPress installation and some dummy content
There’s loads of good tutorials elsewhere on how to set up a WordPress site, and the exact how-to varies slightly according to which hosting provider you use and if they can install it automatically through something like Softaculous. Note that you can’t build and edit a theme like this if you are using a WordPress.com hosted site, but I wouldn’t recommend that route anyway. Grab yourself a domain name and some cheap hosting and you should be set up in 5 minutes or so. Alternatively you can set up a ‘local’ installation on your PC using WAMP or XAMP.
At this point I’d usually add in my most commonly used plugins too. I keep them all in a folder on my PC and drag the whole lot into the wp-content/plugins folder using Filezilla. Included in this is this Dummy content generation plugin Once they’re all in the folder you can find them in your WordPress Dashboard. Most will probably need a 1 click update to the latest version but that only takes a moment. Poplulate your new site with some dummy content (via the Dashboard) so you’ll have something to play with for the rest of the tutorial.
2. Modify the style sheet to register your new WordPress theme
Decide on a theme name (I’ll call mine ‘biscuits’) and then create a new folder in the wp-content/themes one. It will sit alongside the twentytwelve one that comes installed as the WordPress default one nowadays. Now drag in the folders you’ve just downloaded (minus the Photoshop .psd file). Your folder structure should look something like this:
-wp-content
—plugins
—themes
——twentytwelve
——biscuits
———index.html
———portfolio.html
———404.html
———single-blog-post.html
————css
—————-bootstrap.css
—————-bootstrap-responsive.css
———–style.css
———–js
—————-bootstrap-min.js
—————-bootstrap-responsive.-min.js
—————-jquery.pretty-photo.js
—————-jquery.tweet.js
———–img
—————-1.jpg
—————-2.jpg
—————-3.jpg
—uploads
If your theme’s main style sheet is called something else like ‘theme.css’ then you need to rename it as style.css as that’s what WordPress will look for to recognise that there’s a new theme available.
Now make sure that the style.css file is directly in the biscuits folder rather than in a CSS folder within it. Next open the style.css (in Filezilla right-click and select View/Edit – it will open up in your default file editor. Mine is set to Notepad++ for all html, js, css and php files) and copy and paste this CSS comment code at the top
You can change or delete most of the above as long as you keep a Theme Name in there.
In the Flashmint theme I’m working from, the CSS style sheets are loaded separately in the head section like this
I’ve renamed the theme.css file to style.css and put the WordPress comment at the top, Next I’m going to import all those CSS files at the start of style.css (underneath the Theme comments we just added) so it looks something a bit like this.
Make sure your bootstrap-responsive CSS file (if you’re using a responsive version) is always listed after the main bootstrap one as it is supposed to override some of its settings. This theme didn’t come with a reset.css file but if it did I would have imported that at the top of the list.
You can now delete all of those <link href...>
CSS lines from the top of the index.html file.
Save the file and go back to your WordPress Dashboard. Click the Appearance tab and you’ll see that your Biscuits theme is now registered and ready to be enabled. Go ahead and do so if you like, but it won’t be working properly just yet.
3. We’ll take the index.html version (the Home page of the html theme) and turn it into our first WordPress theme template – home.php
WordPress has a hierarchy of templates. None of them are called index (that name is reserved) so we need to rename our index file to something else. Because this is the home page we’ll rename it to home.php – which is the template that WordPress uses for any page or post that is set to be the Home page. Link to codex page about templates.
4. Start with the header
Right, now we’ve got our home.php in our theme folder let’s start editing it so you can change its content easily and automatically from within WordPress.
Here’s the original <head>
section – remember we deleted the style sheets in step 2 so we’ll have to add it back in here.
Let’s add in the title tag so that it is set by WordPress, and add the style.ss file back in. Plus we’ll add in a little line of code that adds the WordPress toolbar to your site so you can edit the content from within the WordPress admin Dashboard.
The Title of the page will now be determined by the Title of your WordPress post or page. Note that the meta description has been removed as that will be inserted by an SEO module where we have fine grain control about its contents.
The <?php bloginfo ?>
is a useful function that grabs some our site’s key attributes like url, name and tagline and makes them available in the templates so that we don’t have to hard code any of that data in. A good thing if we ever want to edit any of these settings.
The wp_head(); line is the magic one that imports all of the WordPress administration functionality into every page and post of your site.
Since these head contents are universal to all of the pages, let’s strip them out and save it in a template called header.php that can then be included in all the other page templates. That way we only need to edit it in one place rather than in each separate template. The Primary menu is also universal to all of the pages so it makes sense to include that in the header.php template too. We’ll hook that up to the WordPress menu system and add our logo later, but for now let’s cut out all of the below code from home.php and paste it in to header.php as well.
Save the contents of the two code boxes above as header.php directly in your theme folder (in my case, in the ‘biscuits’ folder).
If there’s anything I haven’t explained very well, let me know in the comments and I’ll try to clarify it better. In part 2 we’ll move on to the footer…